If you’re like me, you’ve probably felt the challenge of wanting to dive deeper into data science but not knowing where to start. When I first started learning, I found that the best way to understand the concepts was to build something, to see the code come to life and make sense of the data. So, I decided to share 5 Data Science Projects you can tackle this weekend. Each project is designed to be fun, practical, and to help you develop real-world skills. Let’s jump right in!
You can also read more interesting blog post on Data Science and Analytics here
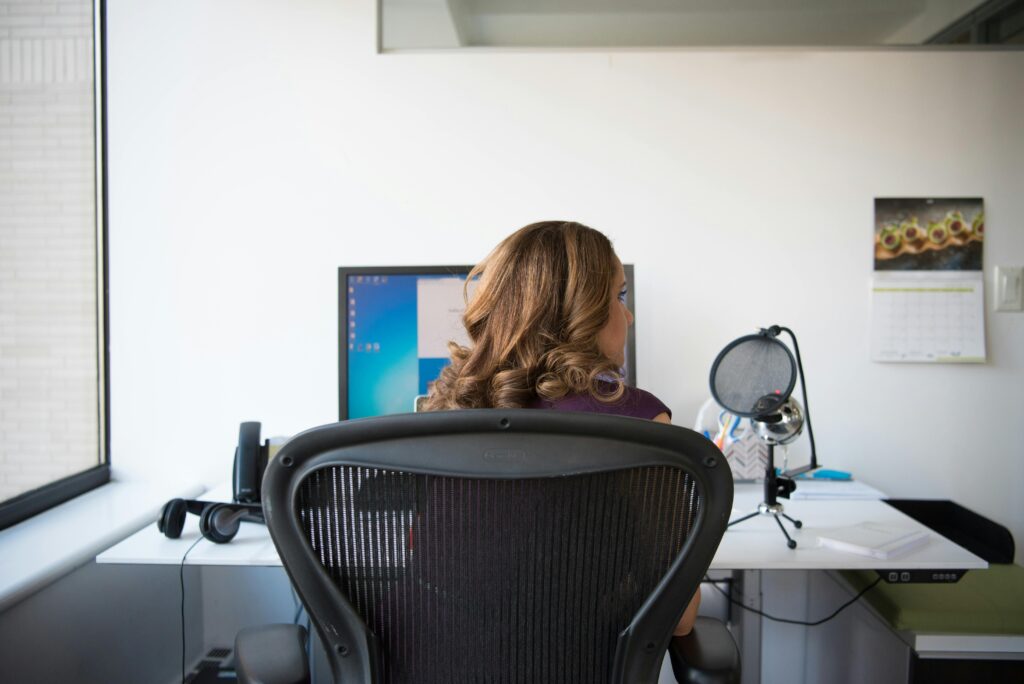
1. Sales Analysis Dashboard (Beginner Level)
Imagine you work for a small retail store, and your manager wants insights into sales performance. Your task is to build a simple dashboard to display key metrics like total sales, average order value, and top-selling products.
Steps to Build
1. Set Up Your Data Use a sample dataset like this:
OrderID,Product,Category,Quantity,Price,Date 1,Sneakers,Footwear,2,50,2025-01-01 2,T-shirt,Clothing,3,20,2025-01-02 3,Jacket,Clothing,1,100,2025-01-03 4,Socks,Accessories,5,5,2025-01-04
2. Load the Data Here’s how you can read the dataset:
import pandas as pd # Load the dataset data = pd.read_csv("sales_data.csv") print(data.head())
3. Analyze Key Metrics Calculate total sales, average order value, and the top product:
# Total sales data['Total'] = data['Quantity'] * data['Price'] total_sales = data['Total'].sum() # Average order value avg_order_value = data['Total'].mean() # Top product top_product = data.groupby('Product')['Total'].sum().idxmax() print(f"Total Sales: ${total_sales}") print(f"Average Order Value: ${avg_order_value}") print(f"Top-Selling Product: {top_product}")
4. Visualize the Data Create bar charts to show sales by category:
import matplotlib.pyplot as plt category_sales = data.groupby('Category')['Total'].sum() category_sales.plot(kind='bar', title='Sales by Category', ylabel='Total Sales', xlabel='Category') plt.show()
By the end of this project, you’ll have a clear dashboard showcasing sales insights, a great addition to your portfolio!
2. Customer Segmentation Using Clustering (Intermediate)
Let’s say you’re working with a marketing team, and they want to identify distinct customer groups. Using clustering techniques like K-Means, you can create segments based on purchase behavior.
Steps to Build
1. Create a Synthetic Dataset Generate mock customer data:
import numpy as np import pandas as pd np.random.seed(42) data = pd.DataFrame({ 'CustomerID': range(1, 101), 'Annual Income (k$)': np.random.randint(20, 120, 100), 'Spending Score': np.random.randint(1, 100, 100) }) print(data.head())
2. Apply K-Means Clustering Group customers into segments:
from sklearn.cluster import KMeans # Select features X = data[['Annual Income (k$)', 'Spending Score']] # Apply K-Means kmeans = KMeans(n_clusters=3, random_state=42) data['Cluster'] = kmeans.fit_predict(X) print(data.head())
3. Visualize the Clusters Use scatter plots to display clusters:
import matplotlib.pyplot as plt for cluster in data['Cluster'].unique(): cluster_data = data[data['Cluster'] == cluster] plt.scatter(cluster_data['Annual Income (k$)'], cluster_data['Spending Score'], label=f'Cluster {cluster}') plt.xlabel('Annual Income (k$)') plt.ylabel('Spending Score') plt.title('Customer Segments') plt.legend() plt.show()
This project helps you practice unsupervised learning and gain insights into customer behavior.
3. Sentiment Analysis Tool (Intermediate)
What are customers saying about your product? A sentiment analysis tool can classify reviews as positive, negative, or neutral. This project is a great introduction to natural language processing (NLP).
Steps to Build
1. Sample Data Use this mock data:
Review,Rating "I love this product!",5 "Terrible experience.",1 "It’s okay, not great.",3
2. Preprocess Text Clean the reviews:
import pandas as pd from sklearn.feature_extraction.text import CountVectorizer data = pd.read_csv("reviews.csv") vectorizer = CountVectorizer(stop_words='english') X = vectorizer.fit_transform(data['Review']) print(vectorizer.get_feature_names_out())
3. Train a Sentiment Classifier Build a model to predict sentiment:
from sklearn.model_selection import train_test_split from sklearn.naive_bayes import MultinomialNB y = data['Rating'] > 3 # Positive sentiment X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) model = MultinomialNB() model.fit(X_train, y_train) print(f"Model Accuracy: {model.score(X_test, y_test)}")
4. Test the Tool Predict sentiment for new reviews:
new_reviews = ["Amazing quality!", "Not worth the money."] X_new = vectorizer.transform(new_reviews) predictions = model.predict(X_new) print(predictions)
With this, you can analyze any text dataset, tweets, reviews, or survey responses.
4. Time Series Forecasting (Advanced)
Forecasting is a core skill in data science. For this project, you’ll predict sales for the next week using historical data.
Steps to Build
1. Prepare the Data Generate mock sales data:
import pandas as pd import numpy as np dates = pd.date_range(start='2025-01-01', periods=30) sales = np.random.randint(50, 200, len(dates)) data = pd.DataFrame({'Date': dates, 'Sales': sales}) print(data.head())
2. Visualize the Trend
import matplotlib.pyplot as plt data.set_index('Date')['Sales'].plot(title='Daily Sales', ylabel='Sales') plt.show()
3. Apply ARIMA
from statsmodels.tsa.arima.model import ARIMA model = ARIMA(data['Sales'], order=(1, 1, 1)) model_fit = model.fit() forecast = model_fit.forecast(steps=7) print(forecast)
By learning forecasting, you’re opening doors to demand prediction and financial analysis.
5. Interactive Visualization App (Advanced)
Turn data into an interactive web app using Streamlit
. You can learn more about Streamlit here!
1. Install Streamlit
pip install streamlit
2. Create a Simple App
import streamlit as st st.title("Sales Dashboard") st.line_chart(data.set_index('Date')['Sales'])
3. Run Your App
streamlit run app.py
These projects are practical, exciting, and perfect for developing your data science skills. Which one will you start this weekend? Let me know in the comments!
You can also check previous post here!